Integrating Meadow into industrial SCADA; Exposing Data over MODBUS TCP
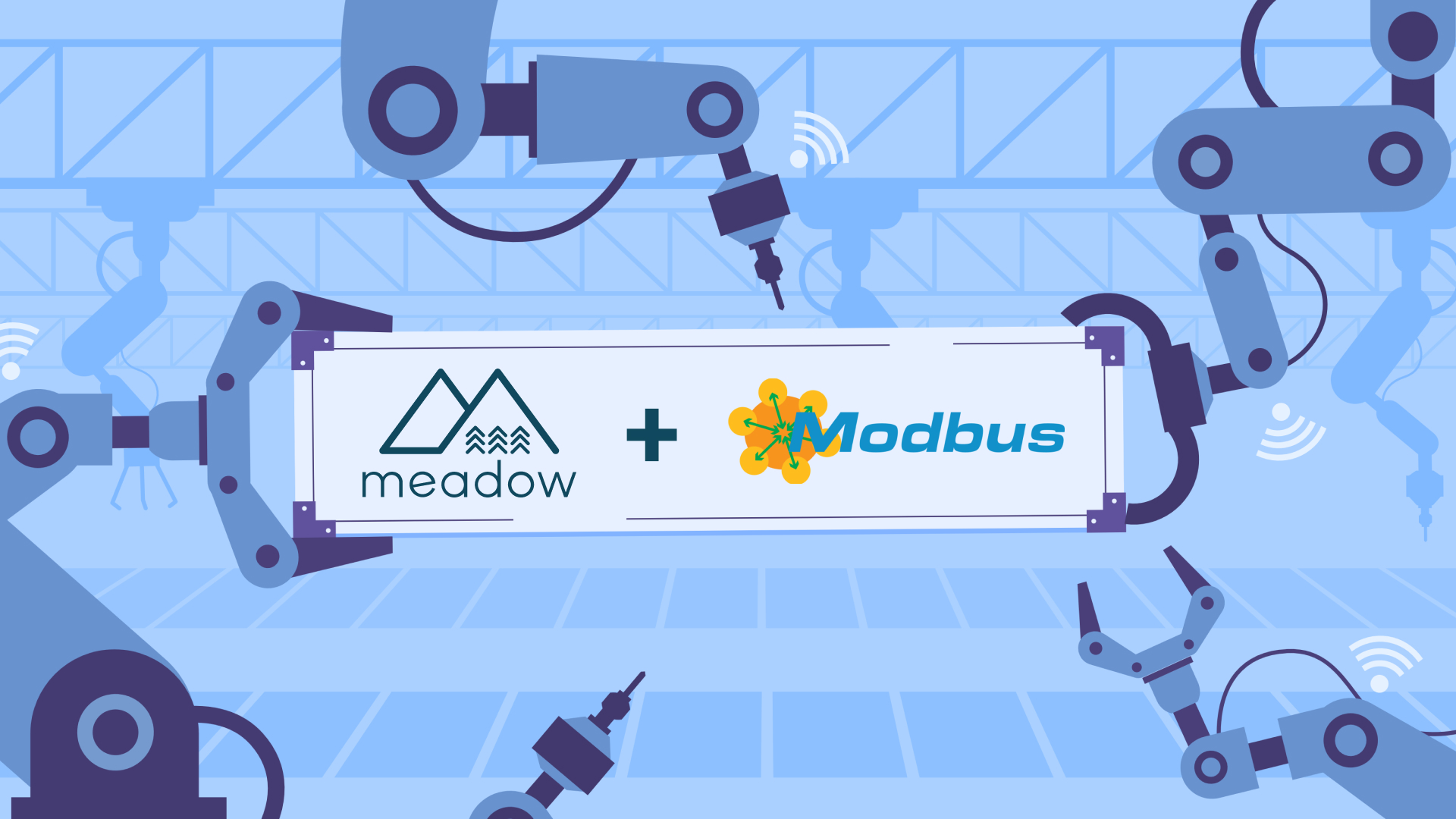
At Wilderness Labs we strive to simplify integrating hardware into your .NET solutions. Industrial automation and process control are very hardware-heavy, and Meadow provides the ability to add in sensors and telemetry to your plant floor in a fast, inexpensive, and unobtrusive way. You can use Meadow device that can monitor machine or environment data and then expose that data for consumption by other machines on the floor.
Industrial automation and process control frequently need disparate hardware to interact, and one common way for systems to share data is by using the Modbus protocol. PLCs and most robots and cobots have the inherent capability to communicate using Modbus over TCP or RS485.
Using the Meadow.Modbus
library, you can easily run a Modbus TCP server on your Meadow hardware. For example, if you wanted to expose the environmental sensor telemetry from a Project Lab board as Modbus registers, you can create a ModbusTcpServer
and then your application can react to clients requesting register or coil data.
In this example, we’ll set up the temperature to be two 16-bit registers starting at Input Register offset 0 (also referred to as address 30001).
private const ushort TemperatureAddress = 0x00;
var modbusServer = new ModbusTcpServer();
modbusServer.ReadInputRegisterRequest += OnModbusServerReadInputRegisterRequest;
modbusServer.Start();
...
private IModbusResult OnModbusServerReadInputRegisterRequest(byte address, ushort startRegister, short length)
{
if(startRegister == TemperatureAddress)
{
var temperature = (float)
projectLab
.EnvironmentalSensor
.Read()
.Result
.Temperature
.Value
.Celsius;
// Modbus registers are 16-bits. We can send back ushorts or bytes
var dataAsBytes = BitConverter.GetBytes(temperature);
return new ModbusReadResult(dataAsBytes);
}
}
With just the short bit of code above, any equipment on the plant floor network can read the Meadow temperature data using a Modbus TCP read of Input Register 0. It can be extended to many sensors, and the data can also be exposed as holding registers or coils, so integrating it into existing processes and work cells is very easy. If you have any questions on using Modbus or using Meadow in industrial applications, feel free to reach out to us on our public Slack channel or directly on our web site.