Motors in Meadow
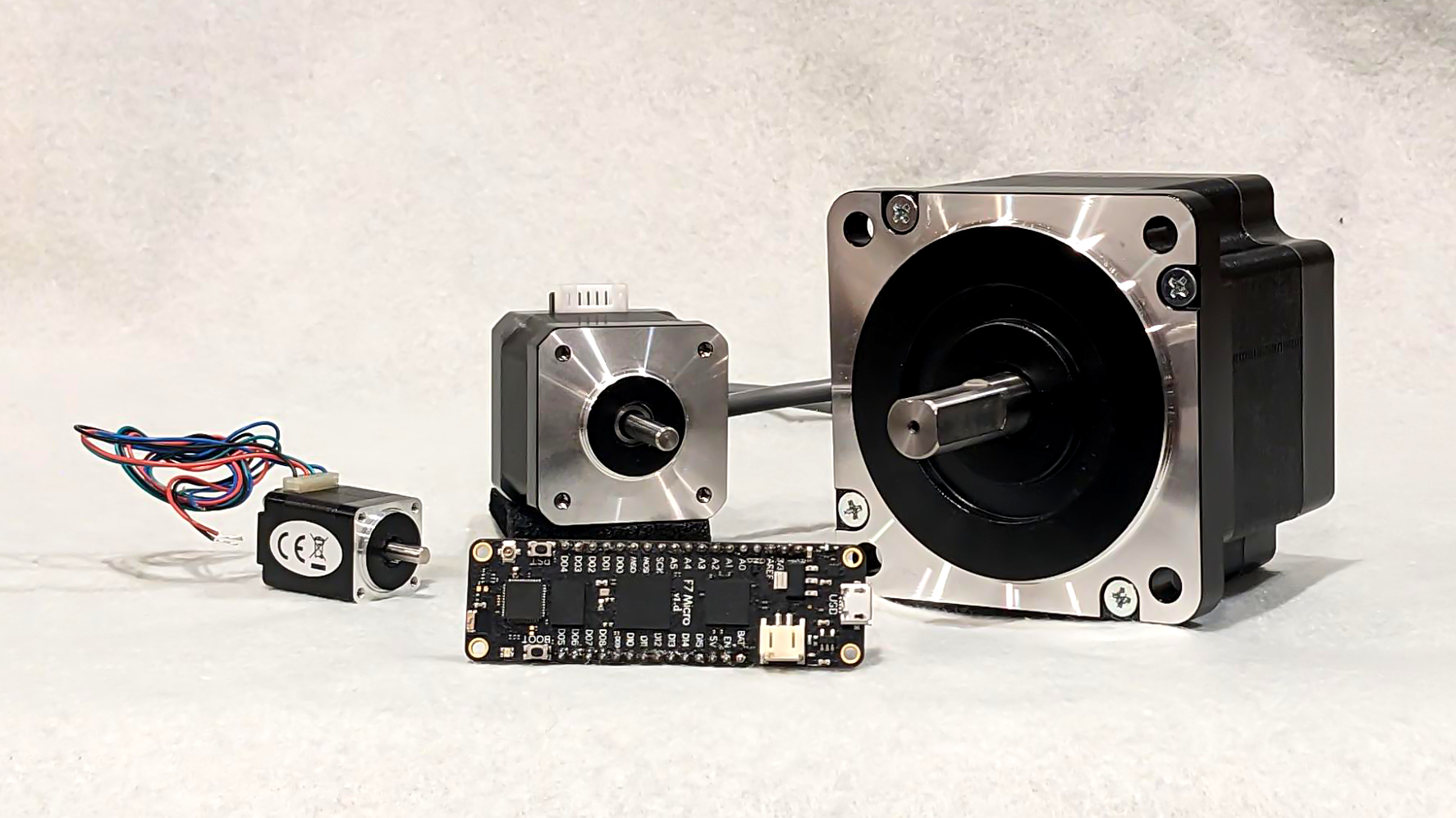
A large subset of what’s called “motion control” is all about using motors to perform actions. From large industrial conveyors and bulk material augers down to 3D printers and hobby robots, motors are everywhere. The question for us is “how do I control those motors with my Meadow?”.
Meadow Motor Interfaces
From a Meadow software perspective, all motors fall into just a few interfaces. In increasing complexity they are: IMotor
, IVariableSpeedMotor
, IPositionalMotor
, and IStepperMotor
.
The IMotor
interface covers the simple capabilities of running a motor in a specified direction and stopping it. A DC motor driving a conveyor belt would be a good example.
The IVariableSpeedMotor
adds, as the name implies, speed to the IMotor
. With it you can command a motor to run at a percentage of its maximum speed. A variable-frequency drive (VFD) is a good example of this sort of motor.
IPositionalMotor
and IStepperMotor
are very similar. The IPositionalMotor
is a motor that you can give very specific positioning commands to, such as calling MoveTo()
with an exact angle and angular velocity. The IStepperMotor
is an IPositionalMotor
that moves to those commanded positions in discrete steps. In the physical world, it’s rare to find an instance of an IPositionalMotor
that isn’t an IStepperMotor
but we’ve separated the interfaces for the occasions where they aren’t.
Using a STEP/DIR Motor
So how do we actually use one of these interfaces from our Meadow application? Let’s look at an example. A very common type of stepper motor drive uses two GPIOs to control the step and direction of the connected motor. This is commonly called a STEP/DIR
motor drive.
You wire up the STEP
and DIR
signals from the drive to the Meadow (be aware that often these will require shifting up from the Meadow’s 3.3V output) and then use the following code to create an instance of the driver:
Var stepper = new StepDirStepper(
Device.Pins.D15.CreateDigitalOutputPort(), // PUL
Device.Pins.D14.CreateDigitalOutputPort(), // DIR
stepsPerRevolution: 200);
You can then, as an example, command to motor to go to the absolute position of 90 degress with the following:
await stepper.GoTo(new Angle(90, UnitType.Degrees),
RotationDirection.Clockwise,
new AngularVelocity(2, UnitType.RevolutionsPerSecond)
Or you can command it to turn a specific number of revolutions like this:
await stepper.Rotate(
new Angle(2.5, Angle.UnitType.Revolutions),
RotationDirection.Clockwise,
new AngularVelocity(2, UnitType.RevolutionsPerSecond)
);
And if you like to control your motor by calculating the number of steps to take yourself, that’s also an option:
await stepper.Rotate(
50, // 50 steps of the motor
RotationDirection.Clockwise,
new Frequency(50, Frequency.UnitType.Hertz)
);
We’ve exposed all of the motor control you might want or need is a simple, straightforward API. So whether you’re doing a small robotics project, or controlling bulk material delivery, rest assured that Meadow can provide the capabilities you need.
Take a look at the full documentation online, or feel free to connect with us on our public Slack channel.