Detecting the Power Source for an F7 Feather
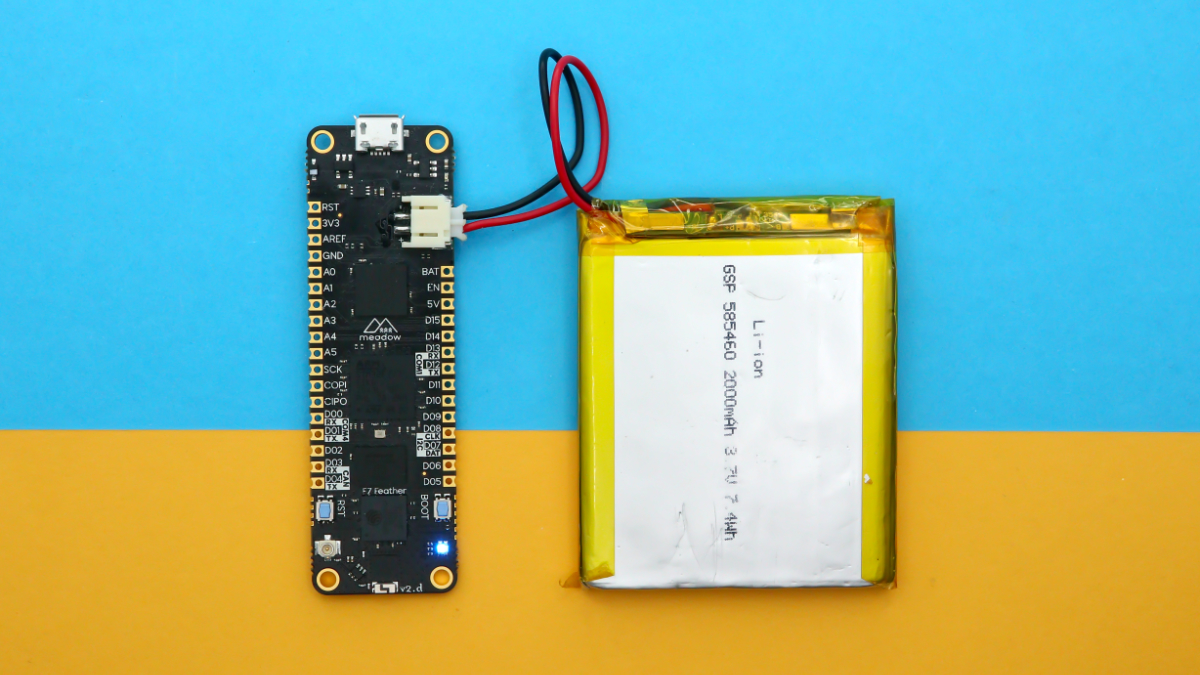
One great feature of our F7 Feather platforms is the built-in battery connector and charging circuit, but developers that use it in their applications almost always follow up with the question of “How can my application know if I’m on line power or battery power?”
There is no inherent circuitry or Meadow.Core
API for detecting the current power source, but adding the capability to your application is pretty simple. The solution relies on the fact that when the Feather is running on battery power, the 5V
rail voltage is significantly below the level when it’s connected to a 5V line source like USB, so your application can simply monitor the actual voltage on the 5V
pin.
Since the Meadow analog inputs’ ability to measure top out at 3.3V (they are 5V tolerant), you can add a simple voltage divider to bring the voltage down. I used an R1
value of 5.6k and an R2
value of 10k. If you monitor the output of this divider with an analog input, if the measured voltage is below 2.75 then the device is running on battery power.
Here’s a simple example that measures the state and adjusts the on-board LED accordingly.
private IAnalogInputPort monitorPort;
private IDigitalOutputPort greenLed;
private IDigitalOutputPort redLed;
public static Voltage PowerThreshold = new Voltage(2.75, Voltage.UnitType.Volts);
public override Task Initialize()
{
monitorPort = Device.Pins.A00.CreateAnalogInputPort(1);
monitorPort.StartUpdating();
greenLed = Device.Pins.OnboardLedGreen.CreateDigitalOutputPort();
redLed = Device.Pins.OnboardLedRed.CreateDigitalOutputPort();
return base.Initialize();
}
public override async Task Run()
{
greenLed.State = true;
while (true)
{
if (monitorPort.Voltage < PowerThreshold)
{
redLed.State = true;
}
else
{
redLed.State = false;
}
await Task.Delay(500);
}
}
And here’s a Fritzing of the setup:
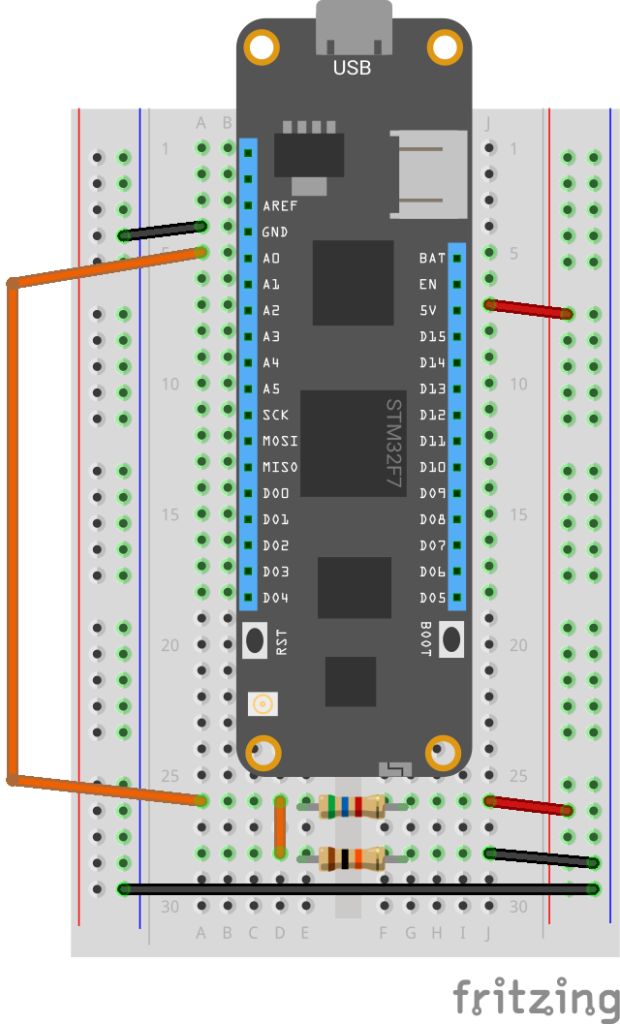
As always, if you have any questions, feel free to contact us over on our public Slack channel.