Hack Kit Series: Closer look at Relays
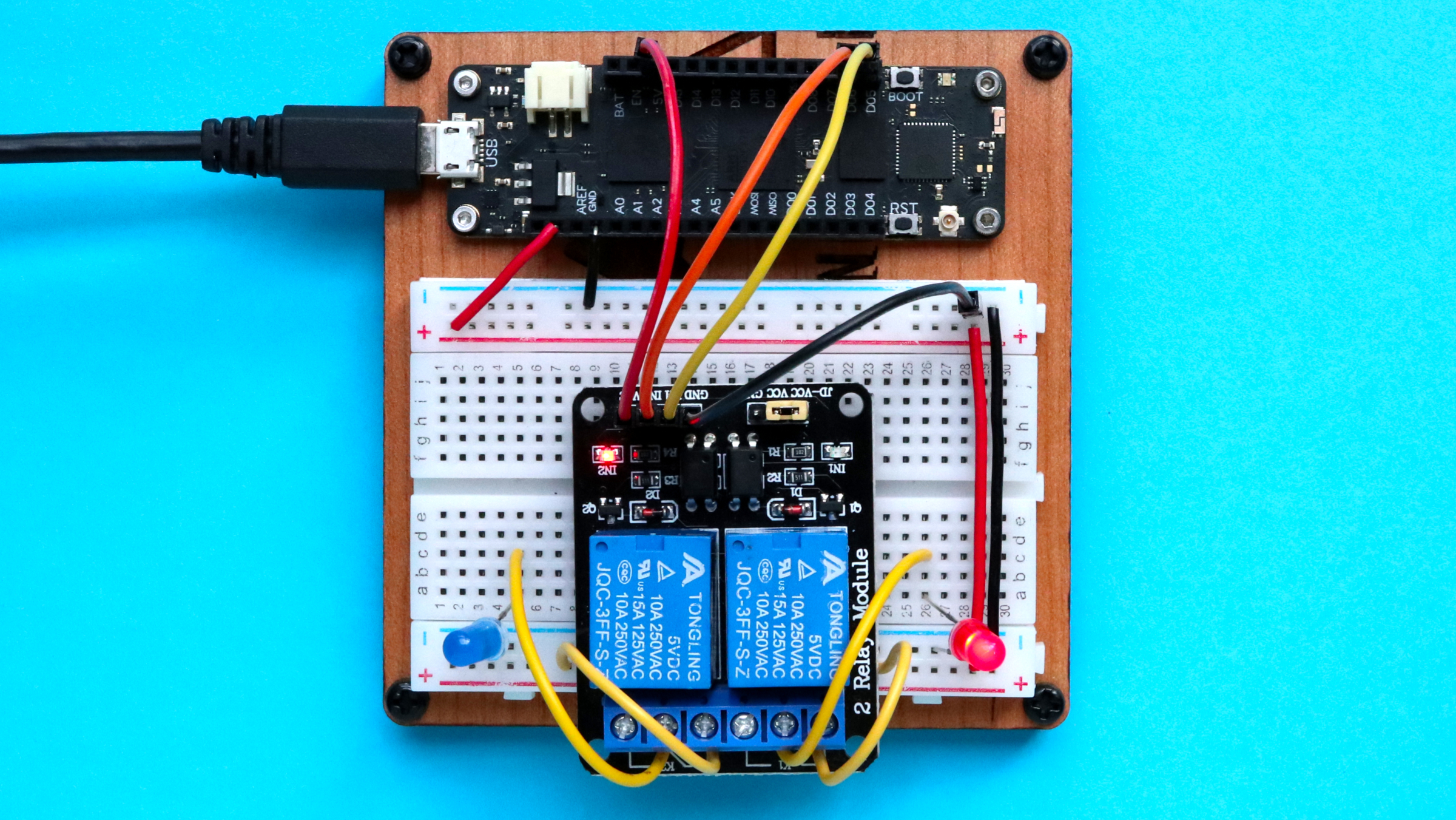
Hello Makers,
It has been a while since I make another post about another peripheral included in Meadow’s Hack Kit. This time we’re going to learn how to work with relays using Meadow.Foundation.
No need to download any NuGet packages to use this peripheral, since this is part of the Meadow.Foundation core library that comes when creating a new Meadow project.
Relays are electromechanical switches that allow a circuit to switch current on another circuit while being electrically isolated from each other. You could typically find these on WIFI-enabled smart plugs so you can control lights or other appliances with your phone, tablet, etc.
Circuit Sample
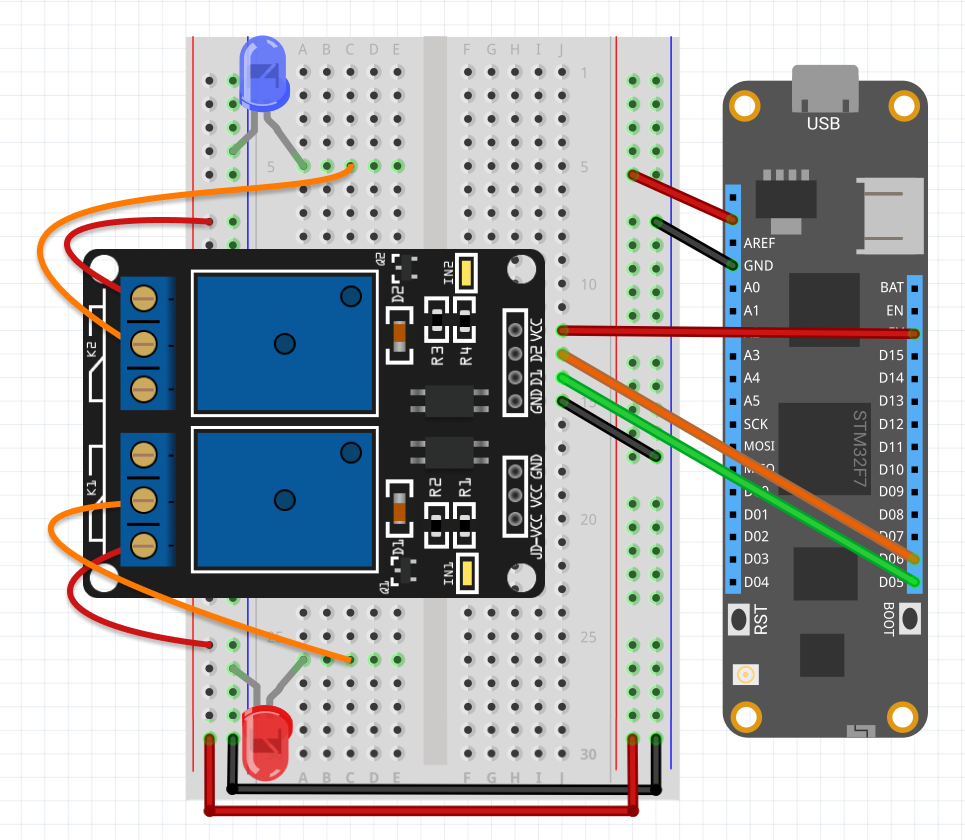
The idea of this circuit is to turn on the LEDs as they were any other peripheral or appliance, connected to GND and 3.3V, with the relay in the middle to act as a switch to turn them on or off.
Code Sample
There’s two ways you can initialize a Relay object:
Option 1
Pass in the Device and Pin connected to the Relay, and the driver will create Digital Output Port internally:
var relayRed = new Relay(Device, Device.Pins.D06);
var relayBlue = new Relay(Device, Device.Pins.D05);
Option 2
Create a Digital Output Port first and pass the port object to the constructor:
var relayRed = new Relay(Device.CreateDigitalOutputPort(Device.Pins.D06));
var relayBlue = new Relay(Device.CreateDigitalOutputPort(Device.Pins.D05));
Usage:
bool state = false;
while (true)
{
state = !state;
Console.WriteLine($"- State: {state}");
relayRed.IsOn = state;
relayBlue.IsOn = !state;
Thread.Sleep(500);
}
In this infinite while loop, notice that we have a boolean state which we alternate in each cycle, and assign it to the relay’s IsOn property to change its value. You should see the Red and Blue led turning on and off, alternating in each cycle.
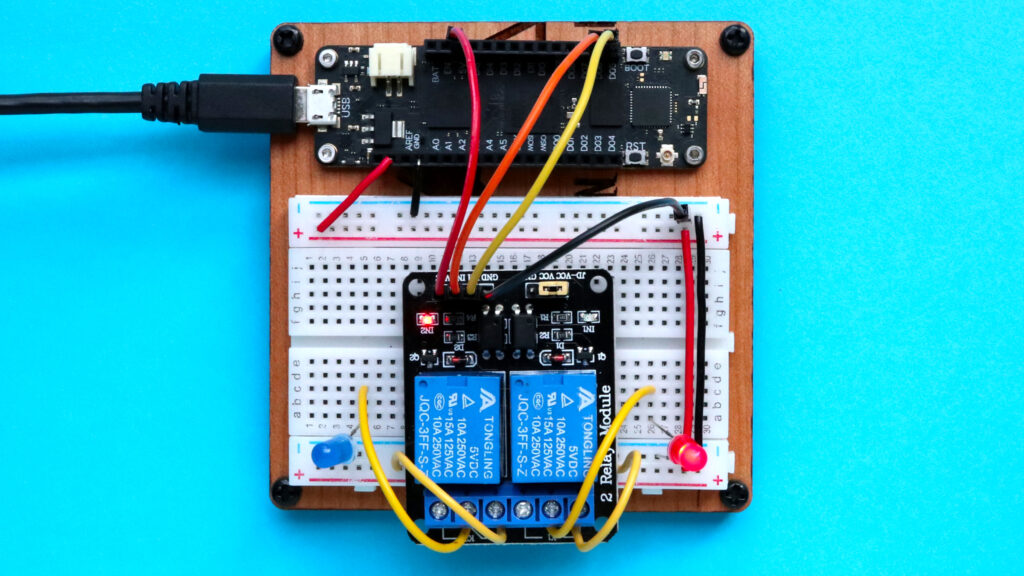
That’s a wrap
This is all I wanted to show you in this quick post. If you want to read more in depth about the Relay API, feel free to check to our official API docs.
Happy hacking and see you on the next post!
Jorge