Hack Kit Series: Closer look at LEDs
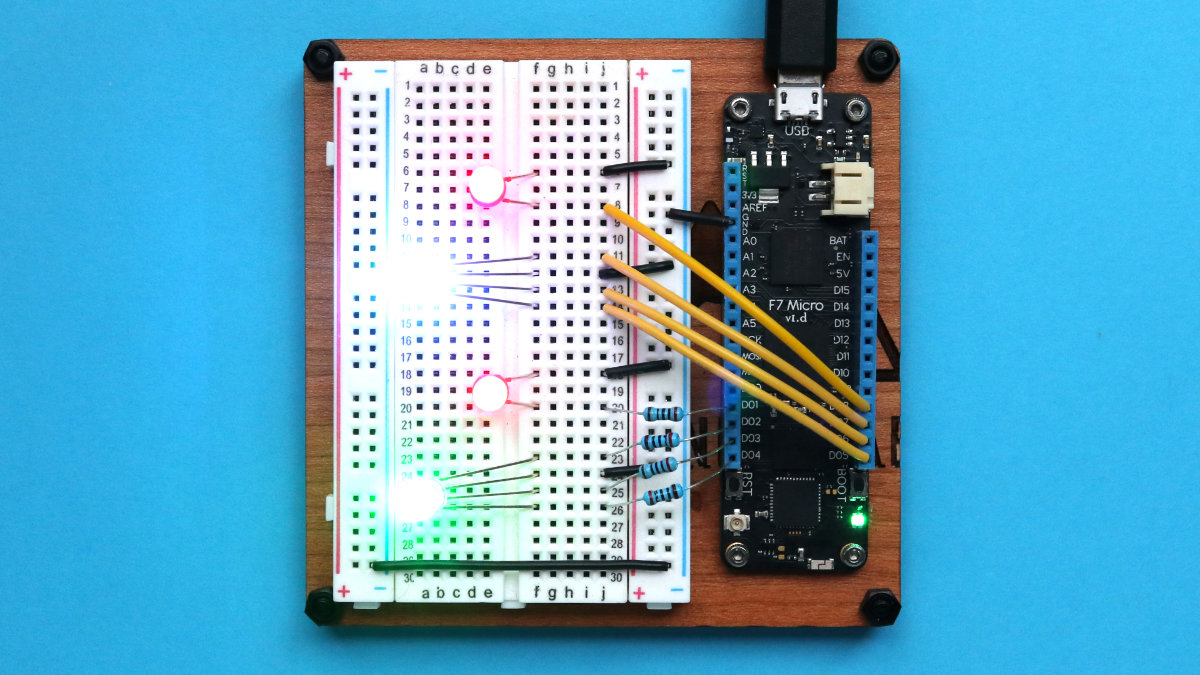
Hello Makers,
In this blog post I want to show you how simple is to control LEDs using Meadow.Foundation. In other platforms you would need to set digital output ports or PWM ports to control the LEDs by changing its values explicitly. With Meadow.Foundation, you only need to instantiate an object specifying to which pin of Meadow the LED is connected, and you can now control the LED with a very intuitive and high level API so you can focus on doing your project than to fiddle with ports and properties to get the animation or behavior you’re hoping to get.
Meadow.Foundation.Leds.Led
Circuit Sample
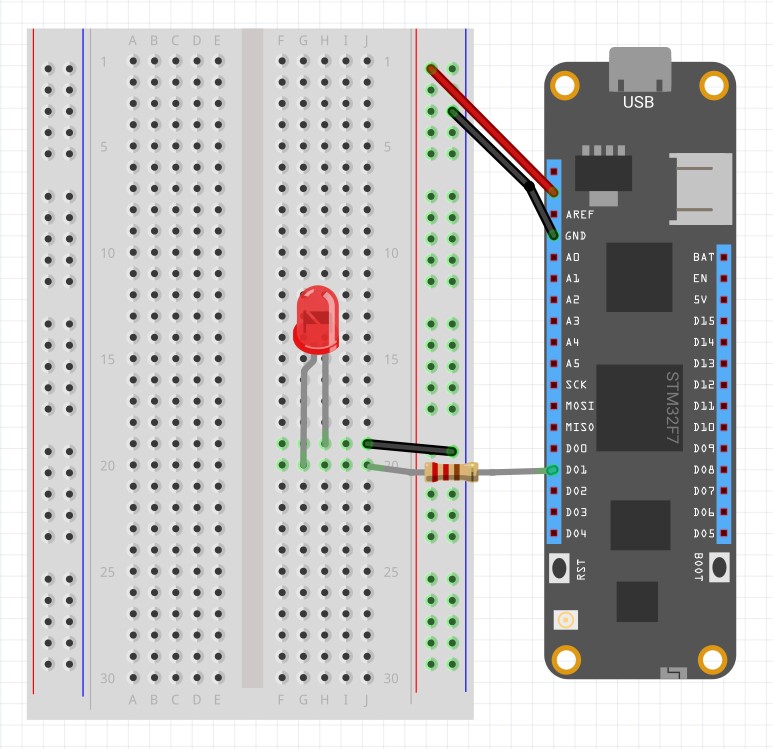
Code Sample
var led = new Led(
pin: Device.Pins.D01
);
//var led = new Led(
//port: Device.CreateDigitalOutputPort(Device.Pins.D01));
led.IsOn = true;
Thread.Sleep(1000);
led.IsOn = false;
To instantiate an Led object, we can either pass the Device object along with the Pin in the constructor so the driver creates the digital output port internally, or we can create the digital output port first and pass them instead.
The Led object provides two basic features:
- IsOn – is a boolean property where you can set it to true or false to turn the LED on or off.
- StartBlink – is a method to make the LED flash on and off at the specified durations expressed in milliseconds.
- Stop – a generic method to stop any Led animations.
Meadow.Foundation.Leds.PwmLed
Circuit Sample
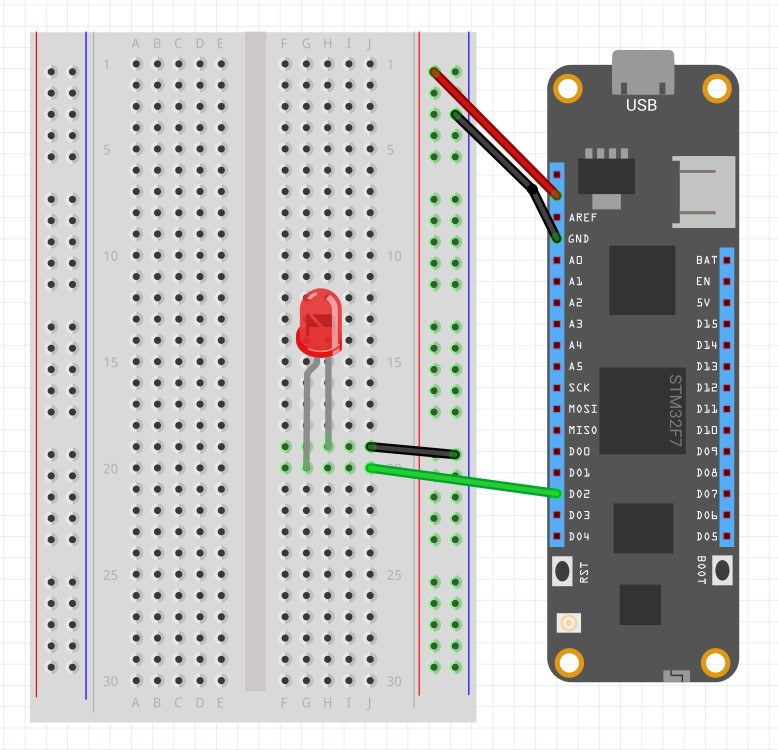
Code Sample
var pwmLed = new PwmLed(
pin: Device.Pins.D01,
TypicalForwardVoltage.Red);
//var pwmLed = new PwmLed(
// pwmPort: Device.CreatePwmPort(Device.Pins.D01),
// TypicalForwardVoltage.Red);
pwmLed.SetBrightness(0.5f);
Thread.Sleep(1000);
pwmLed.Stop();
pwmLed.StartBlink(
onDuration: 500,
offDuration: 500,
highBrightness: 0.75f,
lowBrightness: 0.25f);
Thread.Sleep(1000);
pwmLed.Stop();
pwmLed.StartPulse(
pulseDuration: 1000,
highBrightness: 0.75f,
lowBrightness: 0.25f);
Thread.Sleep(1000);
pwmLed.Stop();
To instantiate a PwmLed object, same as most drivers, we can either pass the Device object along with the Pin in the constructor so the driver creates the PWM port internally, or we can create the PWM port first and pass them instead. Notice we’re also passing in a Forward voltage value so we no longer need to use an external resistor to connect the LED.
The PwmLed has the same basic features the Led has, and have these additional features:
- SetBrightness – is a method to control the level of brightness of the LED, ranging from 0 (off) to 1 (full brightness).
- StartBlink – is a method to make the LED flash on and off at the specified high/low brightness (ranging from 0 to 1) and on/off durations expressed in milliseconds.
- StartPulse – is a method to animate the LED make a pulsing animation at the specified cycle duration and high/low brightness.
- Stop – a generic method to stop any LED animations.
Meadow.Foundation.Leds.RgbLed
Circuit Sample
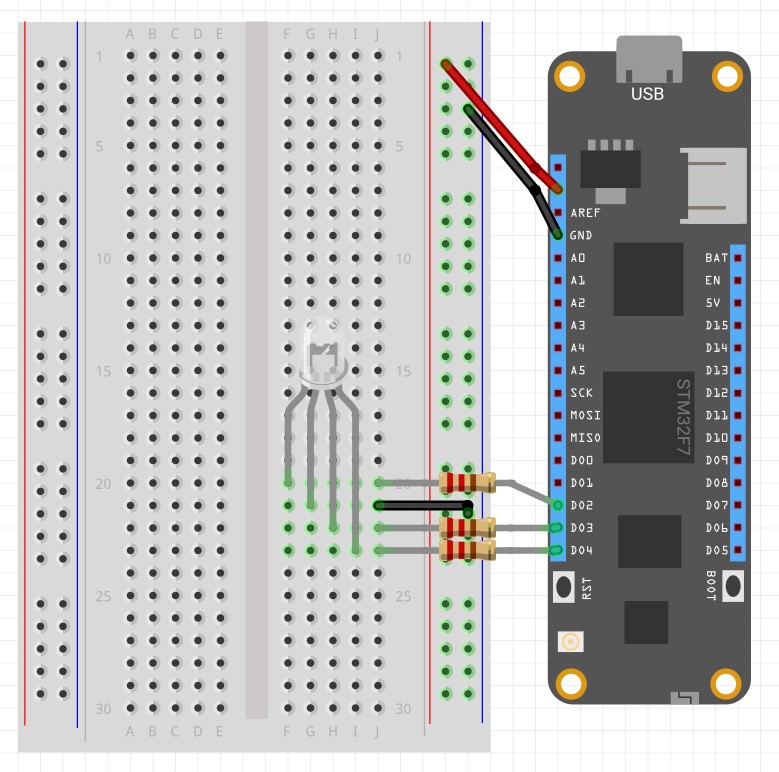
Code Sample
var rgbLed = new RgbLed(
redPin: Device.Pins.D02,
greenPin: Device.Pins.D03,
bluePin: Device.Pins.D04);
//var rgbLed = new RgbLed(
// redPort: Device.CreateDigitalOutputPort(Device.Pins.D02),
// greenPort: Device.CreateDigitalOutputPort(Device.Pins.D03),
// bluePort: Device.CreateDigitalOutputPort(Device.Pins.D04));
rgbLed.IsOn = true;
Thread.Sleep(1000);
rgbLed.IsOn = false;
rgbLed.StartBlink(
color: RgbLed.Colors.Red,
onDuration: 300,
offDuration: 300);
Thread.Sleep(1000);
rgbLed.Stop();
To instantiate an RgbLed object, notice that we can either pass the Device object along with the Pins in the constructor so the driver creates the digital output ports internally, or we can create the digital output ports first and pass them instead.
The RgbLed have the following features, similar to Led but with slight variations:
- IsOn – is a property to turn the RGB LED on or off with the current Color selected.
- SetColor – is a method to turn the RGB LED on in the specified color. Since this driver uses three digital output ports, there’s only up to eight possible combinations (including white when all three are on and black when all three are off).
- StartBlink – is a method to make the RGB LED flash on and off at the specified Color and on/off durations expressed in milliseconds.
- Stop – a generic method to stop any LED animations.
Meadow.Foundation.Leds.RgbPwmLed
Circuit Sample
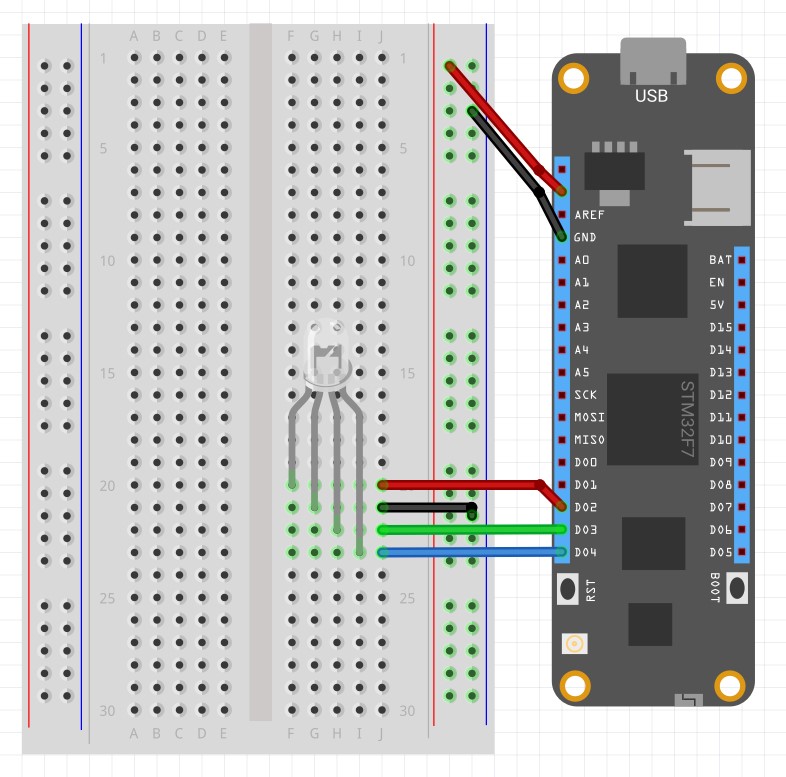
Code Sample
var rgbPwmLed = new RgbPwmLed(
redPwmPin: Device.Pins.D02,
greenPwmPin: Device.Pins.D03,
bluePwmPin: Device.Pins.D04,
redLedForwardVoltage: TypicalForwardVoltage.Red,
greenLedForwardVoltage:TypicalForwardVoltage.Green,
blueLedForwardVoltage: TypicalForwardVoltage.Blue);
//var rgbPwmLed = new RgbPwmLed(
// redPwm: Device.CreatePwmPort(Device.Pins.D02),
// greenPwm: Device.CreatePwmPort(Device.Pins.D03),
// bluePwm: Device.CreatePwmPort(Device.Pins.D04),
// redLedForwardVoltage: TypicalForwardVoltage.Red,
// greenLedForwardVoltage:TypicalForwardVoltage.Green,
// blueLedForwardVoltage: TypicalForwardVoltage.Blue);
rgbPwmLed.SetColor(
color: Color.RoyalBlue,
brightness: 0.25f);
Thread.Sleep(1000);
rgbPwmLed.SetColor(
color: Color.PaleGreen,
brightness: 0.75f);
rgbPwmLed.StartPulse(
color: Color.Orange,
pulseDuration: 500,
highBrightness: 0.75f,
lowBrightness: 0.25f);
To instantiate an RgbPwmLed object, notice that we can either pass the Device object along with the Pins in the constructor so the driver creates the PWM ports automatically, or we can create the PWM ports first and pass them instead. Notice we’re also passing in a Forward voltage values on the red, green and blue channels so we no longer need to use an external resistor to connect the LED. They’re all set to 3.3V by default, which the LED can tolerate, but not recommended for extended periods of time.
The RgbPwmLed has the same basic features the Led has, and have these additional features:
- SetColor – is a method to turn the RGB LED on in the specified color along with a brightness level, ranging from 0 (off) to 1 (full brightness).
- StartBlink – is a method to make the RGB LED flash on and off at the specified color, high/low brightness (ranging from 0 to 1) and on/off durations expressed in milliseconds.
- StartPulse – is a method to animate the RGB LED make a pulsing animation at the specified cycle duration and high/low brightness.
- Stop – a generic method to stop any LED animations.
That’s a wrap!
That’s all I wanted to show you on this post. Be sure to check the official API docs we have available for this awesome peripheral. Last thing I want to mention is you might want to go to Hackster to check these projects that use LEDs:
- Control an LED w/ Digital Output or PWM Ports Using Meadow
- Control an RGB LED w/ Digital Output or PWM ports in Meadow
- Build Your Own Simon Game with Meadow
Happy hacking!
Jorge